0. Overview
Abstract Factory Pattern: is defined as a design pattern that provides an interface for creating a group of related or interdependent objects, allowing the client to access these objects without specifying their concrete classes. This way, clients can obtain various products of the same family at different levels without knowing the specifics of the products’ classes.
The Abstract Factory Pattern is a type of creational pattern and can be considered an advanced version of the Factory Method Pattern. In the Factory Method Pattern, the focus is on one product level structure, while the Abstract Factory Pattern deals with multiple product level structures. When a factory structure can create an entire family of objects across different product level structures, the Abstract Factory Pattern becomes simpler and more efficient compared to the Factory Method Pattern.
- Product Family: Refers to a family of products that are functionally related and belong to different product level structures; it is a group of products produced by the same factory, each belonging to a distinct product level. For example, Huawei’s family includes a phone, laptop, and earphones, while Apple’s family includes a phone, laptop, and earphones. Both families represent three product levels: phone, laptop, and earphones.
- Product Class (Product Level): This refers to a product’s inheritance structure. For instance, if the abstract product is a phone, its subclasses might include Huawei and Apple phones. The abstract phone and its specific brands constitute a product level structure, with the abstract phone as the parent class and specific brand phones as its subclasses.
The diagram below illustrates the relationship between product families and product levels. The horizontal axis represents product levels, while the vertical axis represents product families. There are two product families (Huawei Factory, Apple Factory), each with three product levels (phone, laptop, earphones). By identifying the product family and its level, a product can be uniquely determined.
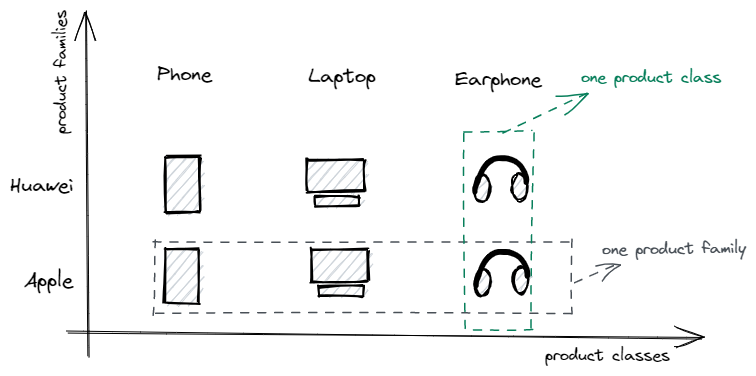
From the diagram above, we can see that each concrete factory can produce all the products belonging to one product family, so the Abstract Factory Pattern only requires two concrete factories. In contrast, using the Factory Method Pattern for six concrete products would require six concrete factories, increasing complexity and the number of classes in the system.
1. Structure and Implementation
Like the Factory Method Pattern, the Abstract Factory Pattern consists of four main elements: abstract factory, concrete factory, abstract product, and concrete product. However, the Abstract Factory Pattern differs in the number of methods within the abstract factory and the number of abstract products.
The main roles are as follows:
- Abstract Factory (IFactory): Provides an interface for creating a series of products, containing multiple product creation methods such as
makePhone()
,makeLaptop()
, andmakeEarphone()
, allowing the creation of products across different levels. - Concrete Factory (HuaweiFactory, AppleFactory): Implements the methods defined in the abstract factory to create specific products within its product family.
- Abstract Product (IProduct, AbstractPhone, AbstractLaptop, AbstractEarphone): Defines product specifications, describing the main characteristics and functions of the products.
- Concrete Product (HuaweiPhone, HuaweiLaptop, HuaweiEarphone, ApplePhone, AppleLaptop, AppleEarphone): Implements the interfaces defined by the abstract product role. Each concrete product is created by a corresponding concrete factory.
The class diagram is as follows:
2. Code Example
2.1. Abstract Products and Concrete Products
Abstract product interfaces and product abstract classes:
1 | // Abstract Product |
Concrete product implementations for the Huawei family:
1 | // Huawei Concrete Earphone Product |
Concrete product implementations for the Apple family:
1 | // Apple Concrete Phone Product |
2.2. Abstract Factory and Concrete Factory
Abstract factory interface:
1 | // Abstract Factory: create different products |
Huawei concrete factory implementation subclass: This class implements the creation of Huawei product family items.
1 | /** |
Apple concrete factory implementation subclass: This class implements the creation of Apple product family items.
1 | /** |
2.3. Usage Example
Example code for using the Abstract Factory pattern:
1 | public class AbstractFactoryMain { |
Execution result:
1 | Create Huawei factory. |
3. Application in Source Code
3.1. JDK - javax.xml.transform.TransformerFactory
TransformerFactory
as an abstract factory defines two abstract methods for creating different objects: Transformer newTransformer()
and Templates newTemplates()
. Its concrete implementation classes, TransformerFactoryImpl
and SmartTransformerFactoryImpl
, override and implement these methods to create the corresponding objects.
3.2. JDK - java.sql.Connection
The java.sql.Connection
interface is analogous to the abstract factory interface, as it provides (produces) different product levels such as Statement
, PreparedStatement
, and CallableStatement
. The products provided are all concrete products under the java.sql.Statement
interface.
1 | public interface Connection extends Wrapper, AutoCloseable { |
Class diagram of the Abstract Factory and its concrete factory implementations:
4. Summary
Applicable Scenarios:
The Abstract Factory pattern was initially applied to create window components for different operating systems, such as the Button and Text components in Java’s AWT, where the native implementations differ between Windows and UNIX. In this case, different systems represent product families, and different components represent product levels.
- A system should not depend on the details of how instances of product classes are created, composed, and represented. This is important for all types of factory patterns.
- When the products to be created are a series of related or interdependent product families, such as the television, washing machine, air conditioner, etc., in an appliance factory.
- When there are multiple product families in the system, but only one family of products is used at a time, meaning only products from a single family are consumed. For example, someone may prefer using products from a particular ecosystem, such as the Xiaomi ecosystem or the Apple ecosystem.
- When the system provides a library of product classes, with all products appearing through the same interface, ensuring that the client is independent of the implementation.
Advantages:
- Separation of Interface and Implementation: The client uses the abstract factory to create the required objects, without knowing the specific implementation. The client programs against the product interfaces, meaning the client is decoupled from the specific product implementations.
- Easy to Switch Product Families: Because a concrete factory implementation represents a product family, for example, switching from the Huawei family to the Apple family only requires replacing the concrete factory.
Disadvantages:
When adding a new factory, such as Xiaomi, you only need to add a new Xiaomi concrete factory class and implement the corresponding product methods, which offers good extensibility. However, when adding a new product, you need to modify the abstract factory, which leads to changes in all factory implementation classes.